RSI Trading Bot Introduction:
Welcome back! In our previous post, we explored backtesting trading strategies with the Relative Strength Index (RSI). Now, let’s take it a step further and build an RSI-based trading bot using Python and the Kotak Neo API.
Algorithmic trading, or algo-trading, has revolted the financial markets by automatically placing trades based on predefined rules. Trading bots, a subset of algo-trading, offer speed, accuracy, and the ability to execute trades without human intervention.
Whether you’re interested in harnessing the power of RSI-based trading strategies, exploring the capabilities of algorithmic trading, or simply curious about building your own trading bot, this tutorial will provide you with the knowledge and tools to embark on your journey into the exciting world of automated trading. So, let’s roll up our sleeves and dive into the fascinating intersection of finance, technology, and automation as we create our very own RSI-based algo trading bot using Python.
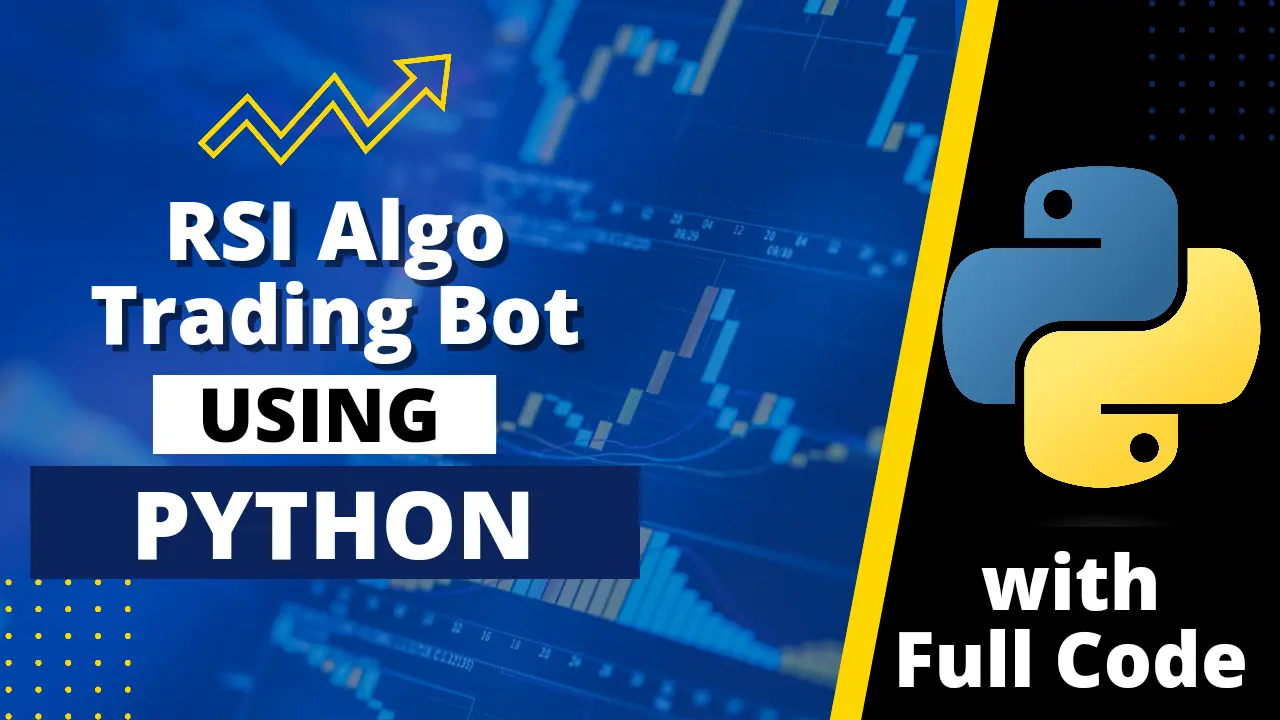
Requirements For Making bots.
To create a trading bot like the one we’re building, you’ll need the following:
- Python: Python provides a versatile and powerful programming language for developing trading bots due to its extensive libraries and ease of use.
- Broker API: We’re using the Kotak Neo API provided by Kotak Neo Broker for accessing market data and executing trades. However, since each user may have a different broker, they’ll need to refer to their broker’s API documentation and make necessary adjustments to the code accordingly.
- WebSocket Data: Continuous and real-time market data is crucial for making timely trading decisions. Utilizing websocket data allows our bot to receive updates on the symbols we’re trading, enabling us to execute buy and sell orders promptly.
- Technical Analysis Library: A library for technical analysis such as TA-Lib and pandas-ta in Python can be helpful for calculating indicators like the Relative Strength Index (RSI) used in our trading strategy.
- Risk Management: Implementing proper risk management techniques is essential for protecting capital and ensuring long-term profitability. This includes setting stop-loss orders, position sizing based on account size and risk tolerance, and monitoring leverage.
- Backtesting Framework: Before deploying our bot in live trading, it’s crucial to backtest our strategy using historical data thoroughly. Utilizing a backtesting framework such as Backtrader or Zipline can help simulate trading performance and identify potential flaws in the strategy. You can use a custom framework also as we used in our Backtesting Rsi trading strategy using Python.
- Security Measures: Since trading bots involve automated execution of trades, ensuring the security of API keys and sensitive information is paramount. Implementing secure practices such as storing API keys securely and utilizing encrypted communication channels is essential to protect against unauthorized access.
By meeting these requirements and customizing the code to suit your broker’s API and individual trading preferences, you’ll be well-equipped to develop and deploy your own automated trading bot. Remember to continuously monitor and evaluate the bot’s performance, making adjustments as necessary to adapt to changing market conditions and optimize profitability.
Historical Data and Symbols:
def get_RSI_Signal_data(symbol, interval="3minute"):
# Calculate end date as the current date and time
end_date = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
# Calculate start date as 1 day before the end date
start_date = (pd.to_datetime(end_date) - pd.Timedelta(days=1)).strftime('%Y-%m-%d %H:%M:%S')
# Assuming historical_data is a function that fetches historical data
# Replace it with your actual function
df = historical_data(symbol, start_date, end_date, interval)
df.rename(columns={'date': 'Date', 'open': 'Open', 'high': 'High', 'low': 'Low', 'close': 'Close', 'volume': 'Volume'}, inplace=True)
# Calculate EMA 20 and EMA 9
df['Rsi'] = ta.rsi(df['Close'], length=14)
df['Atr'] = ta.atr(df['High'],df['Low'],df['Close'], length=14)
df = df.dropna()
# Create a column 'crossover_signal' and initialize it with 0
df['crossover_signal'] = 0
# Find where EMA 9 is above EMA 20 and set 'crossover_signal' to 1
df.loc[(df['Rsi'] > 50) & (df['Rsi'].shift(1) < 50), 'crossover_signal'] = 1
# Find where EMA 9 is below EMA 20 and set 'crossover_signal' to -1
df.loc[(df['Rsi'] < 50) & (df['Rsi'].shift(1) > 50), 'crossover_signal'] = -1
# Convert the 'Date' column to the desired format
df['Date'] = df['Date'].dt.strftime('%Y-%m-%d %H:%M:%S')
# Print the DataFrame with the new 'crossover_signal' column
return df
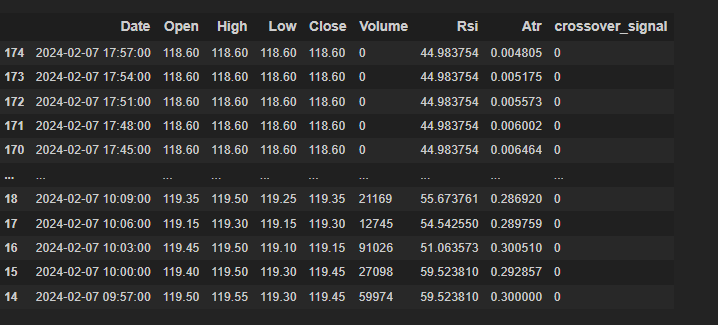
In this function, get_RSI_Signal_data(symbol, interval=”3minute”), we’re leveraging the historical data API provided by the broker to fetch past price data for the specified symbol within a designated time interval. While using the broker’s API for historical data retrieval offers convenience, it comes with certain limitations, notably in terms of speed and responsiveness. Broker APIs may not provide real-time data updates and can be slower in delivering historical data compared to other sources. Consequently, relying solely on broker APIs may result in delayed or incomplete information, which could impact the effectiveness of our trading strategy.
Here’s how the function works:
- It calculates the end date as the current date and time, and then the start date as one day before the end date.
- The function then calls a hypothetical historical_data() function to retrieve historical price data for the specified symbol, start date, end date, and interval. This data is stored in a DataFrame (df).
- The columns of the DataFrame are renamed to match the expected format (‘Date’, ‘Open’, ‘High’, ‘Low’, ‘Close’, ‘Volume’).
- Using the ta library, the function calculates the RSI (Relative Strength Index) and ATR (Average True Range) based on the closing prices.
- It initializes a new column called ‘crossover_signal’ in the DataFrame and sets all values to 0.
- The function identifies points where the RSI crosses above or below the threshold of 50 and sets the corresponding ‘crossover_signal’ values to 1 or -1, respectively, indicating buy or sell signals.
- Finally, it converts the ‘Date’ column to the desired format and returns the DataFrame containing the historical data along with the RSI signals.
To address the limitations of broker APIs and ensure our trading bot operates with the most up-to-date data, an alternative approach involves utilizing websocket data and generating candlesticks in real-time. By doing so, we can overcome the limitations of broker APIs and access more timely and granular insights into market dynamics. If you’re interested in learning how to convert websocket data into candlesticks for dynamic analysis, I’ve previously covered this topic in detail in another blog post, providing step-by-step instructions and code examples. By incorporating websocket data into our trading bot, we can enhance its responsiveness and accuracy, enabling more informed trading decisions and potentially improving overall performance in the market.
Here is How you can convert websocket data to candlesticks.
Getting Live Data:
def get_live_data(symbol):
# Check if the symbol is in the live data dictionary
if symbol in live_data:
return live_data[symbol]
# Handle specific mappings for demo purposes
if symbol == 'YESBANK-EQ' and '11915' in live_data:
return float(live_data['11915'])
elif symbol == 'IDFC-EQ' and '11957' in live_data:
return float(live_data['11957'])
elif symbol == 'GMRINFRA-EQ' and '13528' in live_data:
return float(live_data['13528'])
return None
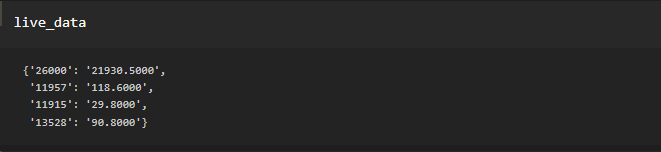
This Python function, get_live_data(symbol), is designed to retrieve live data for a given symbol from a dictionary named live_data. Here’s how it operates:
- Check for Symbol in Dictionary: The function first checks if the symbol is present in the live_data dictionary. If it is, it returns the corresponding data for that symbol.
- Handle Specific Mappings: In case the symbol is not found directly in the live_data dictionary, the function employs specific mappings for certain symbols. This mapping is utilized for demonstration purposes and might be necessary due to variations in how symbols are represented in the data source. For example, if the symbol is ‘YESBANK-EQ’, the function checks if the corresponding token ‘11915’ exists in the live_data dictionary. If it does, it retrieves the data associated with that token and converts it to a float. Similar mappings are applied for other symbols like ‘IDFC-EQ’ and ‘GMRINFRA-EQ’.
- Return None for Unrecognized Symbols: If the symbol is neither directly present in the live_data dictionary nor mapped to a token, the function returns None, indicating that the data for that symbol is not available.
In the context provided, live_data is a dictionary containing continuously updating live data for various symbols, obtained from a websocket feed. Additionally, the function utilizes symbol mappings to ensure compatibility between the symbols used in the code and their representations in the live data dictionary. These symbols represent tradable assets in Indian markets, and users are encouraged to substitute them with symbols relevant to their own trading activities.
Buy and Sell Order Function Through API:
import time
def place_buy_order_with_retry(trading_symbol, quantity):
retry_count = 1 # Number of retry attempts
retry_interval = 5 # Retry interval in seconds
for attempt in range(retry_count):
try:
# Place a Order
buy = client.place_order(
exchange_segment="nse_cm",
product="MIS",
price="",
order_type="MKT",
quantity=str(quantity),
validity="DAY",
trading_symbol=trading_symbol,
transaction_type="B",
amo="NO",
disclosed_quantity="0",
market_protection="0",
pf="N",
trigger_price="0",
tag=None
)
status = get_order_status(buy['nOrdNo'])
st_float = float(status)
if isinstance(st_float, (int, float)):
print(f"Order for {trading_symbol} placed successfully at average price: {status}")
return st_float
else:
print(f"Order not completed for {trading_symbol}. Retrying in {retry_interval} seconds...")
time.sleep(retry_interval)
except Exception as e:
print(f"Exception when calling OrderApi->place_order: {e}")
print(f"Order placement failed for {trading_symbol} after {retry_count} attempts and reason is ")
return False
def place_sell_order_with_retry(trading_symbol, quantity, open_trades):
retry_count = 1 # Number of retry attempts
retry_interval = 5 # Retry interval in seconds
for attempt in range(retry_count):
try:
# Check if symbol is in open trades
if open_trades.get(trading_symbol):
# Place a Sell Order
sell = client.place_order(
exchange_segment="nse_cm",
product="MIS",
price="",
order_type="MKT",
quantity=str(quantity),
validity="DAY",
trading_symbol=trading_symbol,
transaction_type="S",
amo="NO",
disclosed_quantity="0",
market_protection="0",
pf="N",
trigger_price="0",
tag=None
)
status = get_order_status(sell['nOrdNo'])
st_float = float(status)
if isinstance(st_float, (int, float)):
print(f"Order for {trading_symbol} placed successfully at average price: {status}")
return st_float
else:
print(f"Order not completed for {trading_symbol}. Retrying in {retry_interval} seconds...")
time.sleep(retry_interval)
else:
print(f"Symbol {trading_symbol} not found in open trades.")
return False
except Exception as e:
print(f"Exception when calling OrderApi->place_order: {e}")
print(f"Order placement failed after {retry_count} attempts and reason is ")
return False
These two functions, place_buy_order_with_retry(trading_symbol, quantity) and place_sell_order_with_retry(trading_symbol, quantity, open_trades), are responsible for placing buy and sell orders respectively, with retry logic implemented for robustness. Let’s break down each function:
place_buy_order_with_retry(trading_symbol, quantity):
- This function attempts to place a buy order for the specified trading symbol and quantity.
- It defines parameters such as retry_count (number of retry attempts) and retry_interval (retry interval in seconds).
- Inside the retry loop, it tries to place the buy order using the client.place_order() method with the specified parameters.
- If the order placement is successful, it retrieves the order status using get_order_status() and returns the order’s average price as a floating-point number.
- If the order placement fails or encounters an exception, it retries the operation after the specified retry interval.
After exhausting all retry attempts, it prints a failure message and returns False.
place_sell_order_with_retry(trading_symbol, quantity, open_trades):
- Similar to the buy order function, this function attempts to place a sell order for the specified trading symbol and quantity.
- Additionally, it takes an additional parameter open_trades, which is a dictionary containing information about open trades.
- Before attempting to place the sell order, it checks if the symbol exists in the open_trades dictionary. If not found, it returns False.
- Inside the retry loop, it tries to place the sell order using the client.place_order() method with the specified parameters.
- If the order placement is successful, it retrieves the order status using get_order_status() and returns the order’s average price as a floating-point number.
- If the order placement fails or encounters an exception, it retries the operation after the specified retry interval.
After exhausting all retry attempts, it prints a failure message and returns False.
These functions are designed to handle order placement with built-in retry mechanisms to account for potential failures or exceptions during the process, ensuring more robust execution in automated trading scenarios.
Check For StopLoss And Target:
# Function to check for stop-loss and sell conditions for all symbols
def check_stop_loss():
global open_trades
symbols_to_remove = []
for symbol in open_trades.keys():
if stop_loss_condition_met(symbol):
# Close the position
sell = place_sell_order_with_retry(symbol, 1, open_trades)
closed_trade = {
'symbol': symbol,
'entry_price': open_trades[symbol]['entry_price'],
'exit_price': sell,
'pnl': sell - open_trades[symbol]['entry_price']
}
closed_trades.append(closed_trade)
symbols_to_remove.append(symbol) # Reset position after selling
for symbol in symbols_to_remove:
open_trades.pop(symbol, None)
# Function to check for target conditions for all symbols
def check_target():
global open_trades
symbols_to_remove = []
for symbol in open_trades.keys():
if target_condition_met(symbol):
sell=place_sell_order_with_retry(symbol, 1, open_trades)
closed_trade = {
'symbol': symbol,
'entry_price': open_trades[symbol]['entry_price'],
'exit_price': sell,
'pnl': sell - open_trades[symbol]['entry_price']
}
closed_trades.append(closed_trade)
symbols_to_remove.append(symbol) # Reset position after selling
for symbol in symbols_to_remove:
open_trades.pop(symbol, None)
def stop_loss_condition_met(symbol):
live_price = float(get_live_data(symbol))
entry_price = open_trades[symbol]['entry_price']
stop_loss = open_trades[symbol]['stop_loss']
return live_price < stop_loss
def target_condition_met(symbol):
live_price = float(get_live_data(symbol))
entry_price = open_trades[symbol]['entry_price']
target = open_trades[symbol]['target']
return live_price > target
These two functions, check_stop_loss() and check_target(), are responsible for evaluating stop-loss and target conditions respectively for all open trades. Let’s break down each function along with the supporting helper functions:
- check_stop_loss():
This function iterates over all symbols present in the open_trades dictionary to evaluate if the stop-loss condition is met for each symbol. - If the stop-loss condition is met for a particular symbol, it initiates a sell order using place_sell_order_with_retry() function, specifying the symbol, quantity (here assumed as 1), and the open_trades dictionary.
- Upon successful execution of the sell order, it records the details of the closed trade including the symbol, entry price, exit price (derived from the sell order), and profit or loss.
- It then removes the symbol from the open_trades dictionary, effectively closing the position.
check_target():
Similarly to check_stop_loss(), this function iterates over all symbols in the open_trades dictionary to evaluate if the target condition is met for each symbol.
- If the target condition is met for a particular symbol, it initiates a sell order using place_sell_order_with_retry(), specifying the symbol, quantity (1), and the open_trades dictionary.
- Upon successful execution of the sell order, it records the details of the closed trade including the symbol, entry price, exit price (derived from the sell order), and profit or loss.
- It then removes the symbol from the open_trades dictionary, effectively closing the position.
Supporting Helper Functions:
stop_loss_condition_met(symbol): This function checks if the current live price of the symbol is less than the stop-loss price defined for the trade.
target_condition_met(symbol): This function checks if the current live price of the symbol is greater than the target price defined for the trade.
These functions collectively form an integral part of an automated trading system, helping to manage open trades by enforcing predefined stop-loss and target conditions, thus aiding in risk management and profit-taking strategies.
Â
Main Function: Core Rules For Strategy
def main():
global open_trades, closed_trades
while True:
for symbol in symbols:
# Fetch historical data
historical_data = fetch_historical_data(symbol, timeframe='minute')
# Extract the latest signal from the 'crossover' column
latest_signal = historical_data['crossover_signal'].iloc[-1]
print(latest_signal)
#latest_signal = 1
# Execute buy and sell orders based on the latest signal
if latest_signal == 1 and open_trades.get(symbol) is None:
buy = place_buy_order_with_retry(symbol, quantity=1)
if buy == False:
break
entry_price = float(buy)
stop_loss = round((buy*.998),2)
target = round((buy*1.002),2)
open_trades[symbol] = {
'position': 'BUY',
'entry_price': entry_price,
'stop_loss': stop_loss,
'target': target
}
# Check stop-loss and target conditions for all open trades
check_stop_loss()
check_target()
# Print open trades and closed trades for demo purposes
print("Open Trades:", open_trades)
print("Closed Trades:", closed_trades)
print("--------------------")
# Sleep for a specified interval before the next iteration
time.sleep(1) # Sleep for 5 minutes, adjust as needed
# Run the main function
if __name__ == "__main__":
main()
The main() function orchestrates the execution of buy orders based on predefined trading signals in a buy-only strategy. Let’s delve into the functionality of this critical component:
Initialization and Setup:
- The function initializes global variables open_trades and closed_trades, which respectively store information about currently open trades and historical closed trades.
Continuous Execution Loop: - Operating within an infinite loop (while True), the function ensures continuous monitoring and execution of trading operations.
Signal Analysis and Order Execution:
- For each symbol in the list of symbols to trade (symbols), the function fetches historical data and extracts the latest signal from the ‘crossover_signal’ column, indicating a potential buy opportunity.
- If the latest signal is a buy signal (indicated by value 1) and there is no existing open trade for the symbol, a buy order is executed using the place_buy_order_with_retry() function. If the buy order fails, the loop breaks, preventing further execution for the current iteration.
- Upon successful execution of the buy order, the function records the entry price, stop-loss, and target prices for the trade in the open_trades dictionary.
Trade Management: - After executing buy orders, the function continuously monitors for stop-loss conditions for all open trades using the check_stop_loss() function.
- If a stop-loss condition is met for a trade, the corresponding action is executed, and the trade is recorded in the closed_trades list.
Status Display:
- For demonstration purposes, the function prints the current status of open and closed trades at each iteration.
Loop Control and Interval: - To control the frequency of trading activity, the function includes a time delay (time.sleep()) before the start of the next iteration. Adjustments to this interval can be made as needed.
This main() function encapsulates the core logic of the automated trading system, focusing solely on executing buy orders based on predefined signals. When executed, this function operates continuously, providing automated execution of buy-only trading strategies in real-time.
Full Code:
import pandas as pd
import time
import random
# Constants
symbols = ['YESBANK-EQ', 'IDFC-EQ','GMRINFRA-EQ']
# Track current positions and trades
open_trades = {} # Dictionary to track open trades for each symbol
closed_trades = [] # List of dictionaries with closed trades
# Function to fetch historical data (demo, replace with actual data retrieval logic)
def fetch_historical_data(symbol, timeframe):
# Mapping of symbols to actual symbols used for fetching historical data
symbol_mapping = {
"YESBANK-EQ": "YESBANK",
"IDFC-EQ": "IDFC",
"GMRINFRA-EQ": "GMRINFRA"
# Add more mappings as needed
}
# Get the actual symbol from the mapping or use the input symbol if not found
actual_symbol = symbol_mapping.get(symbol, symbol)
# Fetch historical data using the actual symbol
df = get_RSI_Signal_data(actual_symbol, interval=timeframe)[::-1]
return df[0:5]
# Function to simulate fetching live price
def get_live_data(symbol):
# Check if the symbol is in the live data dictionary
if symbol in live_data:
return live_data[symbol]
# Handle specific mappings for demo purposes
if symbol == 'YESBANK-EQ' and '11915' in live_data:
return float(live_data['11915'])
elif symbol == 'IDFC-EQ' and '11957' in live_data:
return float(live_data['11957'])
elif symbol == 'GMRINFRA-EQ' and '13528' in live_data:
return float(live_data['13528'])
return None
# Function to check for stop-loss and sell conditions for all symbols
def check_stop_loss():
global open_trades
symbols_to_remove = []
for symbol in open_trades.keys():
if stop_loss_condition_met(symbol):
# Close the position
sell = place_sell_order_with_retry(symbol, 1, open_trades)
closed_trade = {
'symbol': symbol,
'entry_price': open_trades[symbol]['entry_price'],
'exit_price': sell,
'pnl': sell - open_trades[symbol]['entry_price']
}
closed_trades.append(closed_trade)
symbols_to_remove.append(symbol) # Reset position after selling
for symbol in symbols_to_remove:
open_trades.pop(symbol, None)
# Function to check for target conditions for all symbols
def check_target():
global open_trades
symbols_to_remove = []
for symbol in open_trades.keys():
if target_condition_met(symbol):
sell=place_sell_order_with_retry(symbol, 1, open_trades)
closed_trade = {
'symbol': symbol,
'entry_price': open_trades[symbol]['entry_price'],
'exit_price': sell,
'pnl': sell - open_trades[symbol]['entry_price']
}
closed_trades.append(closed_trade)
symbols_to_remove.append(symbol) # Reset position after selling
for symbol in symbols_to_remove:
open_trades.pop(symbol, None)
def stop_loss_condition_met(symbol):
live_price = float(get_live_data(symbol))
entry_price = open_trades[symbol]['entry_price']
stop_loss = open_trades[symbol]['stop_loss']
return live_price < stop_loss
def target_condition_met(symbol):
live_price = float(get_live_data(symbol))
entry_price = open_trades[symbol]['entry_price']
target = open_trades[symbol]['target']
return live_price > target
# Main trading loop
def main():
global open_trades, closed_trades
while True:
for symbol in symbols:
# Fetch historical data
historical_data = fetch_historical_data(symbol, timeframe='minute')
# Extract the latest signal from the 'crossover' column
latest_signal = historical_data['crossover_signal'].iloc[-1]
print(latest_signal)
#latest_signal = 1
# Execute buy and sell orders based on the latest signal
if latest_signal == 1 and open_trades.get(symbol) is None:
buy = place_buy_order_with_retry(symbol, quantity=1)
if buy == False:
break
entry_price = float(buy)
stop_loss = round((buy*.998),2)
target = round((buy*1.002),2)
open_trades[symbol] = {
'position': 'BUY',
'entry_price': entry_price,
'stop_loss': stop_loss,
'target': target
}
# Check stop-loss and target conditions for all open trades
check_stop_loss()
check_target()
# Print open trades and closed trades for demo purposes
print("Open Trades:", open_trades)
print("Closed Trades:", closed_trades)
print("--------------------")
# Sleep for a specified interval before the next iteration
time.sleep(1) # Sleep for 5 minutes, adjust as needed
# Run the main function
if __name__ == "__main__":
main()
Live Execution Results:
Below are screenshots demonstrating the live execution of the algo trading system described above. These snapshots provide a visual representation of how the code operates in a real-time trading environment. By leveraging this code, you can develop your own personalized trading bots to automate your trading strategies. Feel free to copy and paste the provided code into your machine to experience the results firsthand. These screenshots offer valuable insights into the system’s performance, enabling you to make informed decisions and potentially enhance their trading outcomes. Explore the screenshots below to witness the effectiveness of this automated trading solution in action.
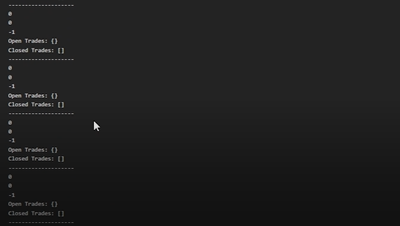
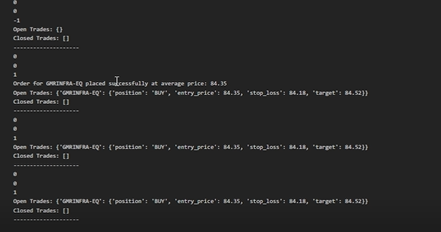
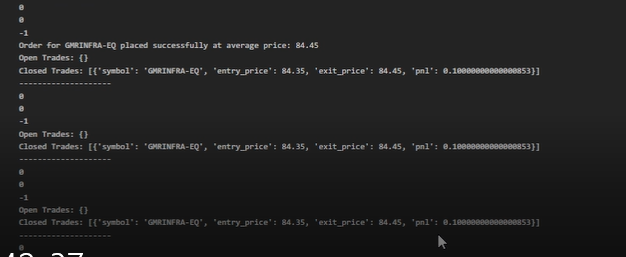
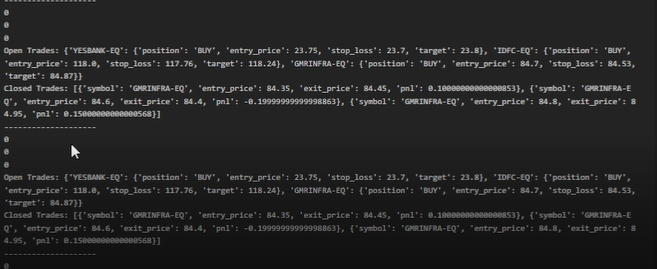
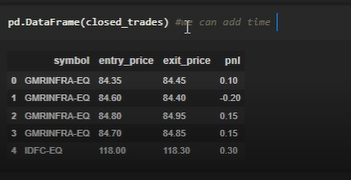
Final Thoughts:
As we conclude the exploration of this buy-only automated trading strategy, it’s essential to acknowledge the inherent risks associated with algorithmic trading. While automated systems offer the potential for increased efficiency and precision in executing trades, they also pose certain risks that traders must consider. One significant risk is the potential for technical failures or errors within the code, which could lead to unintended trade executions or losses. Additionally, market conditions can change rapidly, and algorithmic strategies may struggle to adapt quickly enough, resulting in unexpected outcomes.
Despite these risks, algorithmic trading can provide valuable insights and opportunities for traders. By leveraging historical data analysis and real-time market monitoring, traders can identify and capitalize on potential trading signals more efficiently than manual methods. Furthermore, automated systems can help mitigate emotional biases and human errors that often accompany manual trading, leading to more consistent and disciplined trading decisions.
In conclusion, while algorithmic trading offers promising opportunities for traders to optimize their strategies and streamline their trading processes, it’s crucial to approach automation with a clear understanding of the associated risks and a commitment to diligent risk management practices. With careful planning, testing, and ongoing refinement, traders can harness the power of algorithmic trading to enhance their trading outcomes and achieve their financial goals.